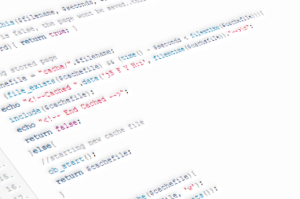
Lets say your website page is already loaded but you need to poll your database for some extra info, or to check for an update on something. The way to go is using Ajax. jQuery makes working with Ajax really easy and even better if you return your data using Json.
Here is some code to get you started with jQuery, Ajax, Json and PHP really fast.
index.html:
<html> <head> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.3.1/jquery.min.js" type="text/javascript"></script> </head> <body> <!-- Here we will display temperature --> <p><label id="temperature">0</label></p> <!-- Here is a link to update temperature --> <p><div id="link">CLICK HERE TO UPDATE</div></p> <script> var city = "NY"; var time = "12:00"; $("#link").click( function(){ var request = $.ajax({ url: "ajax.php", method: "POST", dataType : 'json', data: { city: city, time: time } }); request.done(function( response ) { if( response ['code'] == 1){ $("#temperature").html(response['temperature']); } }); request.fail(function( xhr, textStatus ){ console.log(xhr.responseText); }); } ); </script> </body> </html>
The code above is a simple script. It should update the temperature after clicking the provided link. jQuery listens for the click and starts an ajax request, passed on to ajax.php so it can poll the database with the provided parameters city and time, wich are passed with a POST method.
The variable request will trigger either request.done if success of request.fail if something went wrong when parsing the received Json (usually when the php page outputs an error).
The function request.done will transform the Json response to an array we called ‘response’, so we can access the info in the fashion of an JavaScript array (response[‘mykey’] = mydata).
The function request.fail will output to console the response of the php file in case of fail so it will be easier to debug.
ajax.php:
<?php $city= $_POST['city']; $time = $_POST['time']; $query = "SELECT temperature FROM mytable WHERE city='$city' AND time='$time'"; //your methods for querying and handling the database info here. $response['emperature'] = $mydatabase_response_temperature; $response['code'] = 1; echo json_encode($response, JSON_FORCE_OBJECT); ?>
So that’s it, the second file takes the parameters city and time and performs a query with them to the database. Then you just put the info together in an php array, and echo it converted into json format with json_encode. The parameter JSON_FORCE_OBJECT will give an index to the array in case an index is not specified like in $response[] = $somedata, this will result in JavaScript in a variable response[0] = somedata.
Leave a Reply